Store SDK
A library to save and retrieve secrets and files.
Create a new store and click on the copy icon to get your STORE KEY
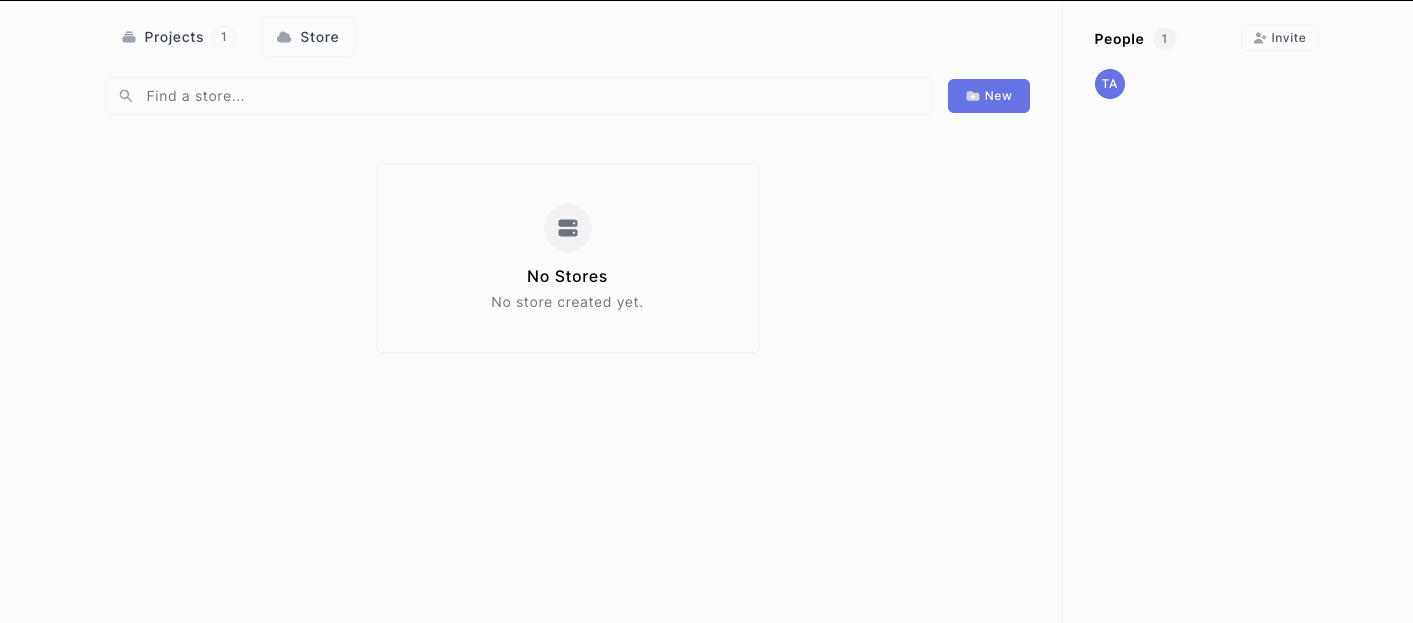
Install
npm install @onboardbase/store
#or
yarn add @onboardbase/store
Initialize the store
import Store from '@onboardbase/store';
const store = new Store('YOUR_STORE_KEY');
APIs
Save a secret with a key
await store.set({ key: 'hey', value: 'hello' });
Get a saved secret with a key
await store.get({ key: 'hey' })
Save a secret with a key within a locker
await store.set({ key: 'hey2', value: 'hello-some-locker', lockerKey: 'some-locker' })
Saves the secret inside of the locker if the locker exists, if the locker doesn't it creates the locker then store the secret within it.
Get a saved secret with a key within a locker
await store.get({ key: 'hey2', lockerKey: 'some-locker' })
Saves a file with a key
const fs = require('fs');
const { Blob } = require('buffer');
let buffer = fs.readFileSync('./.gitignore');
let blob = new Blob([buffer]);
await store.setFile({ key: 'hey-file', fileName: 'gitignore', lockerKey: 'some-locker', file: blob });
// lockerKey is optional, it is created if it doesn't exists before the file is saved
Downloads a file with a key
await store.getFile({ key: 'hey-file', lockerKey: 'some-locker' })
Updated 2 months ago