Secret monitoring
Onboardbase has a feature to monitor secrets in real-time to detect secret leaks. You can use it via webhook or graphical interface.
Via web dashboard
You can view who accessed a secret, when and from where. At the project or organization level.
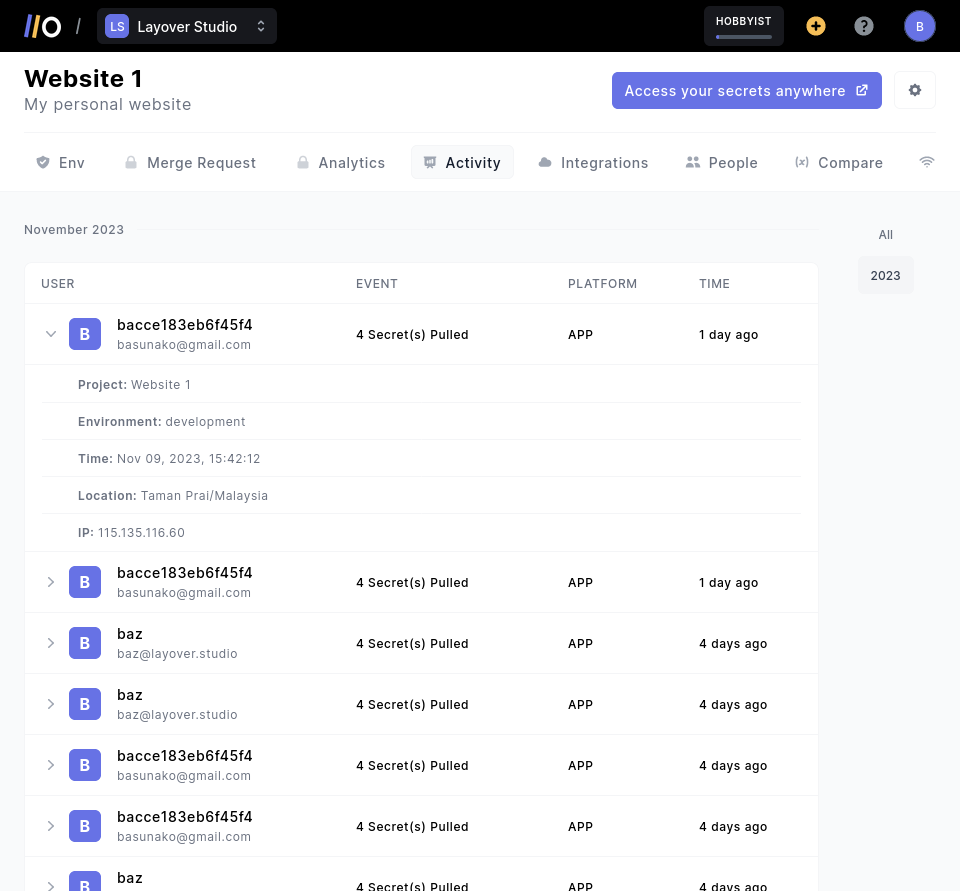
Via webhook
If you want to integrate secret monitoring into your own system, you can use the webhook feature. It will send a POST request to your server every time an event happens.
1. Create a webhook endpoint
You'll need to create a webhook endpoint that can receive POST requests. It should be able to receive JSON data.
You can typically do this using a serverless function or a web framework that creates a http server.
For quick testing, you can visit https://webhook.site to generate a free webhook URL.
2. Create an Onboardbase webhook
Log in to your Onboardbase dashboard, select the project you want to configure webhooks for, then click on the webhooks tab.
From the webhooks page, click on the “Add webhook” button, which pops up a modal asking you to enter your webhook name, URL endpoint, and the environment/s you want to receive events for.
3. Receive events
You'll now receive events in your webhook endpoint:
We currently raise the following events, the list will continue to grow as we extend our webhook functionality.
Event | Description |
---|---|
ENVIRONMENT_UPDATED | An environment's name was updated |
SECRETS_UPDATED | A secret stored on Onboardbase has been updated |
SECRETS_CREATED | A new secret was added, we include the ID of the new secret in the payload |
SECRETS_DELETED | A secret was deleted, we include the ID of the affected secret in the payload |
SECRETS_UPSERTED | A secret was added or updated from one of our integrations: Vercel, Netlify, Heroku |
The JSON payload of the events will look like this:
{
"team": { "name": "Docmini" },
"project": {
"name": "dockerized-nodejs-application",
"description": "A simple dockerized Application",
"createdAt": "2023-03-28T06:29:12.611Z"
},
"environment": {
"title": "development",
"createdAt": "2023-03-28T06:29:12.611Z"
},
"eventType": "ENVIRONMENT_UPDATED"
}
{
"team": {
"name": "Docmini"
},
"project": {
"name": "dockerized-nodejs-application",
"description": "A simple dockerized Application",
"createdAt": "2023-03-28T06:29:12.611Z"
},
"environment": {
"title": "development",
"createdAt": "2023-03-28T06:29:12.611Z"
},
secretIds: ["id1"],
"eventType": "SECRETS_UPDATED"
}
{
"team": {
"name": "Docmini"
},
"project": {
"name": "dockerized-nodejs-application",
"description": "A simple dockerized Application",
"createdAt": "2023-03-28T06:29:12.611Z"
},
"environment": {
"title": "development",
"createdAt": "2023-03-28T06:29:12.611Z"
},
secretIds: ["id1"],
"eventType": "SECRETS_CREATED"
}
{
"team": {
"name": "Docmini"
},
"project": {
"name": "dockerized-nodejs-application",
"description": "A simple dockerized Application",
"createdAt": "2023-03-28T06:29:12.611Z"
},
"environment": {
"title": "development",
"createdAt": "2023-03-28T06:29:12.611Z"
},
secretIds: ["id1"],
"eventType": "SECRETS_DELETED"
}
{
"team": {
"name": "Docmini"
},
"project": {
"name": "dockerized-nodejs-application",
"description": "A simple dockerized Application",
"createdAt": "2023-03-28T06:29:12.611Z"
},
"environment": {
"title": "development",
"createdAt": "2023-03-28T06:29:12.611Z"
},
secretIds: ["id1", "id2"],
"eventType": "SECRETS_UPSERTED"
}
4. (optional) Verifying Webhook Signatures
You can verify the events that Onboardbase sends to your webhook endpoints. Alongside the event body we send, we also include a x-onboardbase-signature
Header in the webhook events.
A HMAC SHA512
signature of the event payload signed with your Signing Secret
is the value of this header. Before processing any event, the header signature should be checked.
const express = require("express");
const crypto = require("crypto");
const app = express();
app.use(express.json());
function GenerateSignature(secret, body) {
const stringifyBody = JSON.stringify(body);
return crypto
.createHmac('sha512', secret)
.update(stringifyBody)
.digest('hex');
}
const signingSecret = process.env.SIGNING_SECRET;
app.post("/webhook", async (req, res) => {
const signature = req.headers["x-onboardbase-signature"];
const generatedSignature = GenerateSignature(signingSecret, req.body);
// check that the signature is valid before processing the event
if(signature === generatedSignature) {
// process the webhook event
}
res.json({
status: "success",
message: "Webhook received",
});
});
const port = process.env.PORT
app.listen(port, () => {
console.log(`Webhook listening on port ${port}`);
});
Updated about 1 year ago